C syntax Wikipedia
Table Of Content

Further, the kernel itself (at least in the case of Linux) operates independently of any libraries. The most common C library is the C standard library, which is specified by the ISO and ANSI C standards and comes with every C implementation (implementations which target limited environments such as embedded systems may provide only a subset of the standard library). This library supports stream input and output, memory allocation, mathematics, character strings, and time values. Several separate standard headers (for example, stdio.h) specify the interfaces for these and other standard library facilities. Functions may be written by the programmer or provided by existing libraries.
Operator precedence
We have improved the exposition of critical features, such as pointers, that are central to C programming. We have refined the original examples, and have added new examples in several chapters. For instance, the treatment of complicated declarations is augmented by programs that convert declarations into words and vice versa. As before, all examples have been tested directly from the text, which is in machine-readable form. Heap memory allocation has to be synchronized with its actual usage in any program to be reused as much as possible. For example, if the only pointer to a heap memory allocation goes out of scope or has its value overwritten before it is deallocated explicitly, then that memory cannot be recovered for later reuse and is essentially lost to the program, a phenomenon known as a memory leak.
Arrays
It does not include a standard set of "container types" like the C++ Standard Template Library, let alone the complete graphical user interface (GUI) toolkits, networking tools, and profusion of other functionality that Java and the .NET Framework provide as standard. The main advantage of the small standard library is that providing a working ISO C environment is much easier than it is with other languages, and consequently porting C to a new platform is comparatively easy. Unions in C are related to structures and are defined as objects that may hold (at different times) objects of different types and sizes.
Selection statements
The constants may be used outside of the context of the enum (where any integer value is allowed), and values other than the constants may be assigned to paint_color, or any other variable of type enum colors. However, the built-in functions must behave like ordinary functions in accordance with ISO C. The main implication is that the program must be able to create a pointer to these functions by taking their address, and invoke the function by means of that pointer. If two pointers to the same function are derived in two different translation units in the program, these two pointers must compare equal; that is, the address comes by resolving the name of the function, which has external (program-wide) linkage. Yes, your microcontroller registers are in fact pointers to a hard-coded memory address. If you take a look at the Register summary section in the ATmega’s data sheet, you will find those two registers are mapped indeed to addresses 0x24 and 0x25 respectively. C++ also contains the type conversion operators const_cast, static_cast, dynamic_cast, and reinterpret_cast.
Thus, a do statement always executes its sub-statement at least once, whereas while may not execute the sub-statement at all. Though logically the last subscript in an array of 10 elements would be 9, subscripts 10, 11, and so forth could accidentally be specified, with undefined results. The primary facility for accessing the values of the elements of an array is the array subscript operator. To access the i-indexed element of array, the syntax would be array[i], which refers to the value stored in that array element. The POSIX standard added several nonstandard C headers for Unix-specific functionality. A number of other groups are using other nonstandard headers – the GNU C Library has alloca.h, and OpenVMS has the va_count() function.
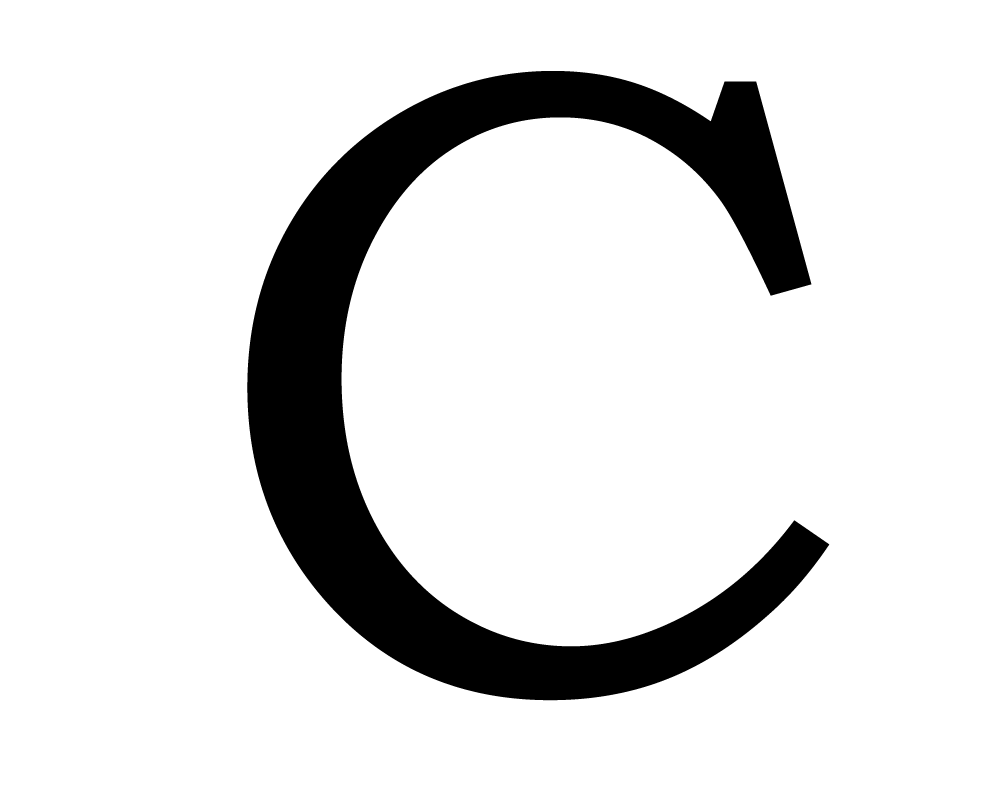
For more precise specification of width, programmers can and should use typedefs from the standard header stdint.h. Three of the header files (complex.h, stdatomic.h, and threads.h) are conditional features that implementations are not required to support. A number of tools have been developed to help C programmers find and fix statements with undefined behavior or possibly erroneous expressions, with greater rigor than that provided by the compiler. Another common set of C library functions are those used by applications specifically targeted for Unix and Unix-like systems, especially functions which provide an interface to the kernel.
The C standard library in other languages

(Although char can represent any of C's "basic" characters, a wider type may be required for international character sets.) Most integer types have both signed and unsigned varieties, designated by the signed and unsigned keywords. Signed integer types may use a two's complement, ones' complement, or sign-and-magnitude representation. In many cases, there are multiple equivalent ways to designate the type; for example, signed short int and short are synonymous. The next line calls (diverts execution to) a function named printf, which in this case is supplied from a system library.
The difference is that "A" represents a null-terminated array of two characters, 'A' and '\0', whereas 'A' directly represents the character value (65 if ASCII is used). The same backslash-escapes are supported as for strings, except that (of course) " can validly be used as a character without being escaped, whereas ' must now be escaped. The use of other backslash escapes is not defined by the C standard, although compiler vendors often provide additional escape codes as language extensions. One of these is the escape sequence \e for the escape character with ASCII hex value 1B which was not added to the C standard due to lacking representation in other character sets (such as EBCDIC). An incomplete type is a structure or union type whose members have not yet been specified, an array type whose dimension has not yet been specified, or the void type (the void type cannot be completed).
Treatment for deadly superbug C. diff may be weakening - Medical Xpress
Treatment for deadly superbug C. diff may be weakening.
Posted: Thu, 25 Apr 2024 16:50:02 GMT [source]
C syntax
Obituary: Yvette C. Poulin - Kennebec Journal and Morning Sentinel
Obituary: Yvette C. Poulin.
Posted: Fri, 26 Apr 2024 05:01:01 GMT [source]
C identifiers are case sensitive (e.g., foo, FOO, and Foo are the names of different objects). Some linkers may map external identifiers to a single case, although this is uncommon in most modern linkers. The main function will usually call other functions to help it perform its job.
This specifies most basically the storage duration, which may be static (default for global), automatic (default for local), or dynamic (allocated), together with other features (linkage and register hint). This is a perfectly valid cast, and as long as accessing members of bar won’t go beyond the size of foo, we will be on the safe side. Whether such a type cast makes sense or not is of course a different story, and depends on the context, but pointers give us the freedom to cast as we please. Another way to use this freedom is assembling sequential data to one common buffer.
The width of the int type varies especially widely among C implementations; it often corresponds to the most "natural" word size for the specific platform. The standard header limits.h defines macros for the minimum and maximum representable values of the standard integer types as implemented on any specific platform. Void pointers are commonly found in dynamic memory allocation functions, for example as return type for malloc() and as parameter type for free(), and any other generic memory accessing functions such as memcpy() or fwrite(). Keep in mind though, since it removes details of the data itself, dereferencing a void pointer will result in a compiler error, unless we first cast to an explicit pointer type. A consequence of C's wide availability and efficiency is that compilers, libraries and interpreters of other programming languages are often implemented in C.[45] For example, the reference implementations of Python,[46] Perl,[47] Ruby,[48] and PHP[49] are written in C. These three approaches are appropriate in different situations and have various trade-offs.
According to the C standard the macro __STDC_HOSTED__ shall be defined to 1 if the implementation is hosted. An implementation can also be freestanding which means that these headers will not be present. If an implementation is freestanding, it shall define __STDC_HOSTED__ to 0.
Because they are typically unchecked, a pointer variable can be made to point to any arbitrary location, which can cause undesirable effects. In general, C is permissive in allowing manipulation of and conversion between pointer types, although compilers typically provide options for various levels of checking. Some other programming languages address these problems by using more restrictive reference types. Unix-like systems typically have a C library in shared library form, but the header files (and compiler toolchain) may be absent from an installation so C development may not be possible. The C library is considered part of the operating system on Unix-like systems; in addition to functions specified by the C standard, it includes other functions that are part of the operating system API, such as functions specified in the POSIX standard.
For example, static memory allocation has little allocation overhead, automatic allocation may involve slightly more overhead, and dynamic memory allocation can potentially have a great deal of overhead for both allocation and deallocation. C89 is supported by current C compilers, and most modern C code is based on it. Any program written only in Standard C and without any hardware-dependent assumptions will run correctly on any platform with a conforming C implementation, within its resource limits. Except the extreme case with gets(), all the security vulnerabilities can be avoided by introducing auxiliary code to perform memory management, bounds checking, input checking, etc. This is often done in the form of wrappers that make standard library functions safer and easier to use. Pike where the authors commonly use wrappers that print error messages and quit the program if an error occurs.
Most compilers issue a warning if there is more than one such pair, though. String literals may not contain embedded newlines; this proscription somewhat simplifies parsing of the language. To include a newline in a string, the backslash escape \n may be used, as below. The benefit to using the second example is that the numeric limit of the first example isn't required, which means that the pointer-to-array could be of any size and the second example can execute without any modifications. This syntax produces an array whose size is fixed until the end of the block. The largest allowed array subscript is therefore equal to the number of elements in the array minus 1.
Comments
Post a Comment